How Do Coroutine Work In Unity?
Agnesh Pipaliya
Jul 14, 2022
236
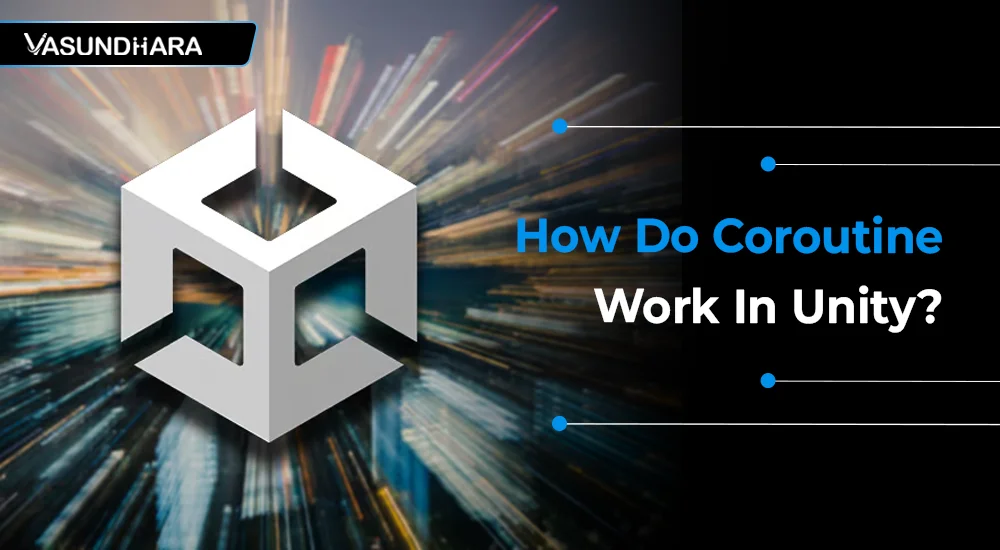
While programming in Unity, you try to delay a function or wait for another function to finish, and sometimes you want to pause it halfway to queue up a series of actions to take place one after another. You have found it difficult to perform all this with an Update function. But this is the situation where Coroutines come into action.
What Is a Coroutine?
A coroutine is like a function that can pause its execution and return control to the same point after a condition is met.
As we know, simple functions can return any type, whereas coroutines must return an IEnumerator, and we must use "yield" before "return". This is the main difference between standard C# functions and coroutine functions.
Before we take a jump to coroutine workflow, you should understand why it is required. And that is what makes it a powerful feature that can do wonders in Unity.
Why Do We Need a Coroutine In Unity?
We already know that the Unity platform is frame-based and it does things each frame. When we call a function, it runs to completion before returning. This effectively means that any action taking place in a function must happen within a single frame update; a function call can not be used to contain a procedural animation or a sequence of events over time; it is also not able to pause its execution. This is where the coroutines come in very handy. Coroutines give us full control of how we want to finish this task.
Usually, coroutines are used to break up workflow into multiple frames. You might be thinking we can do this using the Update function in Unity, but we do not have any control over the Update function, whereas coroutine code can be executed at a different frequency (for example, every 3 seconds instead of every frame).
Syntax
Generally, coroutine function looks as shown below image:
When we use yield, the execution of the code is paused and resume later, depending on which type “yield” used.
Yield return null – This “yield” has ability to resumes execution after All Update functions have been called, on the next frame.
Yield return new WaitForSeconds(t) – This “yield” has ability to resumes execution after (Approximately) t seconds.
Yield return new WaitForSecondsRealtime(t) - This “yield” has ability to suspends the coroutine execution for the given amount of seconds using unscaled time.
Yield return new WaitForEndOfFrame() – This “yield” has ability to resumes execution after all cameras and GUI were rendered.
Yield return new WaitForFixedUpdate() – This “yield” has ability to resumed execution after all FixedUpdates have been called on all scripts.
Yield return new WWW(URL) – This “yield” has ability to resumes execution after the web resource at URL was downloaded or failed.
How To Start And Stop Coroutine?
There are two types of methods to start Coroutine, both ways using the StartCoroutine() function.
IEnumerator Based : StartCoroutine(IEnumerator e) eg. StartCoroutine(MySampleCoroutine())
String Based : StartCoroutine(string name of function) eg. StartCoroutine(“MySampleCoroutine”)
String-based are cause to error and this only works for coroutines that have no input parameters. If your coroutines have any input parameter you must be used IEnumerator based. So it recommended using IEnumerator based coroutines.
StartCoroutine(): In your code, where you want to stop or wait for the execution of some code you just call a method name that has an IEnumerator return type in StartCoroutine function. See below image for more clarification.
StopCoroutine(): Stopping Coroutine using this method(IEnumerator based) is hard, because if the same Coroutine has been called more than once using StartCoroutine(IEnumerator abc) method, then StopCoroutine(IEnumerator abc) do not any reference to which one to stop. It might stop the one which is not desired.
The best way is to save the reference of the IEnumerator before starting the coroutine. See below image for more clarification.
Types Of Coroutines
Basically Coroutines method is used 3 types:
- Asynchronous Coroutines
- Synchronous Coroutines
- Parallel Coroutines
Asynchronous Coroutines
Unity allows us to start new coroutines within an existing coroutine. The most simple way in which this can be achieved is by using StartCoroutine. When invoked, the spawned coroutine co-exist in parallel with the original one. They do not interact directly, and most importantly they do not wait for each other.
Note: In this example, “MyCoroutine_B” is a totally independent coroutine. Terminating MyCoroutine_A does not affect MyCoroutine_B and vice versa.
Synchronous Coroutines:
It is also possible to execute a nested coroutine and to wait for its execution to be completed. The simplest way to do this is by using “yield return”.
It’s worth noting that, since the execution of MyCoroutine_A is suspended during the execution of MyCoroutine_B, this particular case does not need to start another coroutine.
Parallel Coroutines:
It is also possible to execute a nested coroutine and to wait for its execution to be completed. The simplest way to do this is by using “yield return”.
When a coroutine is started using StartCoroutine, a special object is returned. This can be used to query the state of the coroutine and, optionally, to wait for its termination.
In the example below the coroutine, MyCoroutine_B is executed asynchronously. Its father MyCoroutine_B can continue its execution for as long as it needs. Then, if necessary, it can yield to the reference to MyCoroutine_B for an asynchronous wait.
Conclusion:
A Coroutine is the most useful function of Unity, as you can see in this blog. Therefore, you need to learn this function to develop engaging and effective game applications for your clients.