How to Add In-App Purchases in Unity Games
Ronak Pipaliya
May 21, 2025
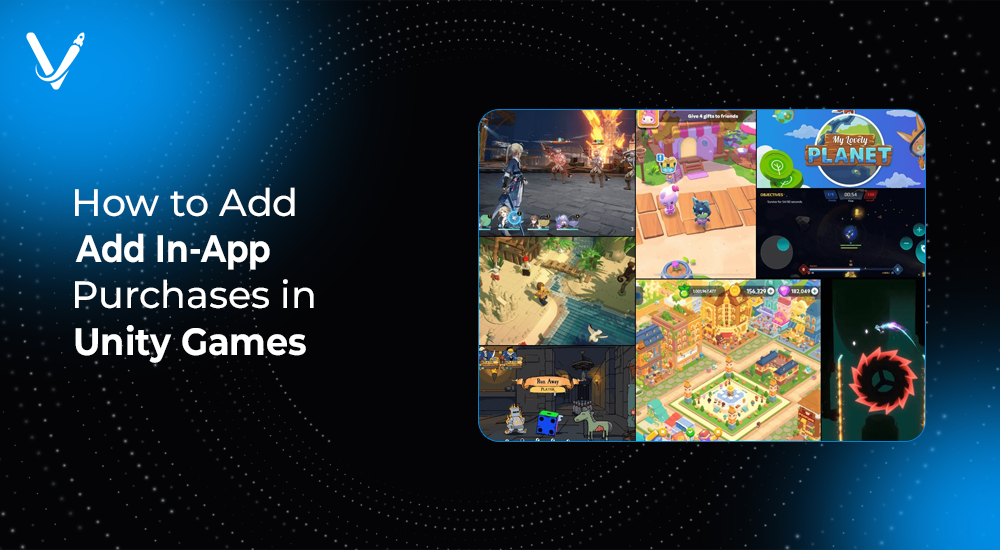
Monetization is a crucial aspect of modern game development, especially in mobile and free-to-play models. Among the most effective monetization strategies is in-app purchasing, which allows players to buy digital items, upgrades, and virtual currency within the game. If you’re a developer working with Unity, learning how to integrate Unity IAP (In-App Purchasing) is essential to generating revenue and enhancing user experience.
This guide walks you through every aspect of adding in-app purchases to Unity games—from setup to best practices—along with real-world examples, code snippets, and actionable insights. Whether you're building for iOS, Android, or multiple platforms, this article will equip you with everything needed to implement monetization effectively using Unity.
Why In-App Purchases Matter in Unity Games
As mobile gaming continues to dominate app stores, monetization models are evolving. In-app purchases have emerged as one of the most profitable methods, especially for free-to-play titles. In 2025, over 75% of mobile game revenue comes from IAPs, surpassing ads and subscriptions in many cases.
Benefits of Adding In-App Purchases in Unity Games:
- Scalable Revenue Model: Earn recurring income by offering consumables, non-consumables, and subscriptions
- Enhanced Player Engagement: Virtual goods, skins, boosters, and features create deeper engagement
- Cross-Platform Support: Unity IAP supports Android, iOS, macOS, and Windows, simplifying deployment
- Seamless Integration: Unity provides a built-in system for adding IAP functionality, saving time and reducing reliance on third-party SDKs
If you’re working with a Unity game development company or building independently, knowing how to work with Unity IAP is a key skill in today’s mobile gaming ecosystem.
Understanding Unity IAP and Unity Gaming Services
Unity IAP is a service provided by Unity Gaming Services (UGS) that allows developers to easily implement in-app purchases across platforms using a unified API. It simplifies the complex process of managing multiple app store integrations.
Unity IAP Supports:
- Google Play Store
- Apple App Store
- Windows Store
- Mac App Store
Unity Gaming Services (UGS) also provides tools for analytics, remote configuration, and player engagement, making it easier to manage and scale monetization strategies.
Types of In-App Purchases You Can Add
Before diving into implementation, it's important to understand the different types of IAPs:
Consumables
Items that are purchased, used, and can be purchased again (e.g., in-game currency, health potions)
Non-Consumables
One-time purchases that unlock permanent content or features (e.g., premium skins, extra levels)
Subscriptions
Recurring payments that unlock ongoing benefits or content (e.g., battle passes, VIP access)
Setting Up Unity IAP in Your Project
Step 1: Prepare Your Unity Project
Ensure your Unity version is up to date (preferably Unity 2021 or later). Start by creating a new project or opening your existing game
- Go to Window > Package Manager
- Install the In-App Purchasing package
- Enable Unity IAP through the Services window (Window > Services)
Unity Gaming Services must be set up and linked with your Unity project ID.
Step 2: Create IAP Products in Stores
Define your IAP items on the Google Play Console or Apple App Store Connect. For each item, configure:
- Product ID (must match what you use in Unity)
- Pricing (localize if possible)
- Type: Consumable, Non-consumable, or Subscription
- Description and Icon
Example:
- Product ID: com.yourgame.coinpack1
- Type: Consumable
- Price: $0.99
Step 3: Enable Unity IAP in Code
In your Unity project, create a script to initialize Unity IAP and define available products.
csharp
CopyEdit
using UnityEngine.Purchasing;
public class IAPManager : MonoBehaviour, IStoreListener
{
private static IStoreController storeController;
private static IExtensionProvider extensionProvider;
public static string COIN_PACK = "com.yourgame.coinpack1";
void Start()
{
var builder = ConfigurationBuilder.Instance(StandardPurchasingModule.Instance());
builder.AddProduct(COIN_PACK, ProductType.Consumable);
UnityPurchasing.Initialize(this, builder);
}
public void OnInitialized(IStoreController controller, IExtensionProvider extensions)
{
storeController = controller;
extensionProvider = extensions;
}
public void BuyCoinPack()
{
storeController.InitiatePurchase(COIN_PACK);
}
public PurchaseProcessingResult ProcessPurchase(PurchaseEventArgs args)
{
if (args.purchasedProduct.definition.id == COIN_PACK)
{
Debug.Log("Coin pack purchased!");
// Add coins to player's wallet
}
return PurchaseProcessingResult.Complete;
}
public void OnPurchaseFailed(Product product, PurchaseFailureReason failureReason)
{
Debug.LogError("Purchase failed: " + failureReason);
}
}
Step 4: Test In-App Purchases
Use the Google Play testing tracks (internal, alpha, or beta) or TestFlight for iOS. Ensure testers are registered, and your build is uploaded properly. Testing must use real accounts and sandbox environments provided by Apple and Google.
Best Practices for Implementing IAP in Unity
Use Clear Product Descriptions
Make sure players understand what they are purchasing. Use visual cues like icons, tooltips, and popups.
Balance Game Economy
Ensure items are valuable but not pay-to-win. Maintain a balance to avoid alienating non-paying users.
Offer Limited-Time Deals
Use Unity Gaming Services’ Remote Config to dynamically create discounts, bundles, or time-sensitive offers.
Protect Against Fraud
Store receipts and verify purchases server-side when possible to prevent spoofing or refund exploits.
Localize for Global Reach
Use localized pricing, descriptions, and currencies to increase conversion in international markets.
Real-World Example: Monetizing a Casual Mobile Game
Imagine a mobile puzzle game with 10 million downloads. The developer implements Unity IAP with:
- Coin Packs (consumables): Used for hints and level skips
- Ad Removal (non-consumable): A one-time upgrade
- Premium Skins (non-consumable): Visual upgrades for in-game characters
- Monthly Subscription: Unlocks exclusive levels and early access
By analyzing player behavior using Unity Analytics and A/B testing different pricing models, the developer increases ARPU (average revenue per user) by 18% in 6 months, all while keeping the core gameplay free.
Integrating IAP with Unity Gaming Services
Use Unity’s Remote Config to control which products are visible at what times. This allows you to test which in-app offers perform best in different regions or user groups.
Pair IAP with Unity Analytics to track:
- Conversion rates
- Average transaction value
- Retention post-purchase
Send in-game events (e.g., iap_purchase_success) to analyze user journeys and refine your monetization funnel.
Common Mistakes to Avoid
Using inconsistent product IDs across stores and Unity code
- Forgetting to test purchases on real devices
- Not validating receipts, leaving room for hacks
- Neglecting UI/UX, leading to confusion or accidental purchases
- Overpricing items, which reduces conversion and increases uninstall rates
What’s Next for Unity IAP in 2025?
As mobile and cross-platform gaming grow, Unity continues to enhance its Unity IAP service. Expect new features like:
- Subscription management dashboards
- Built-in analytics integrations
- Personalized offers via machine learning
- Deeper integration with ads and engagement tools
Whether you’re a solo dev or a Unity game development company, the opportunity to create profitable games with well-designed in-app purchases has never been better.
Conclusion: Monetize Your Unity Game the Smart Way
Adding in-app purchases to your Unity game is not just about revenue—it's about creating a more engaging and rewarding experience for players. With Unity IAP and Unity Gaming Services, you can seamlessly implement consumables, non-consumables, and subscriptions across platforms. By following best practices and testing strategies, you ensure that monetization enhances gameplay rather than disrupts it.
At Vasundhara Infotech, we help developers and studios build, scale, and monetize games using cutting-edge tools and practices. Whether you need help integrating Unity IAP or building a complete game, our experts are ready to assist.
Contact us today to turn your Unity game into a successful business.