How To Navigate From One View Controller To Another Using Swift?
Somish Kakadiya
Jun 27, 2022
224
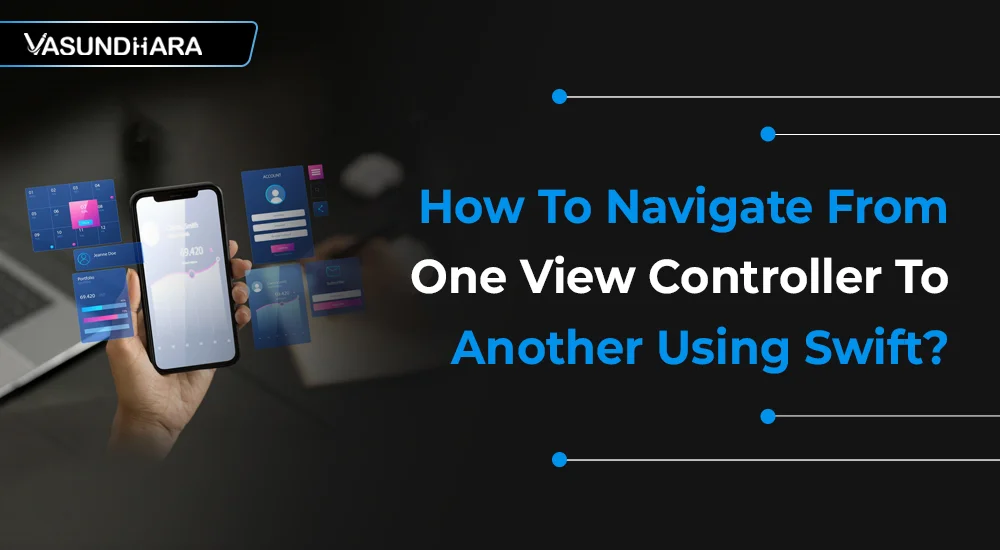
A Navigation Controller is a special kind of view controller that managed a stack of view controllers and their corresponding views.
Navigation Controller allows you to manage a large number of view controllers in a stack.
Navigation Controllers view is consist of many subviews that include a navigation bar at the top.
It contains the optional title, back button, and other bar buttons.
How To Add Navigation Controller In Your Project
There are some simple steps and its necessary to add into your project:
- Step - 1: Select the initial view controller in your storyboard.
- Step - 2: Go to Editor -> Embed In -> Navigation Controller.
Switch between View Controller is really important for every application.
You can switch between View Controller with three options:
1. Segue
2. Push / Pop
3. Present / Dismiss
1. Segue
Segue is a connection between two view controllers and scene.
There are many types of segues.
1. Show (e.g.: Push Segue)
2. Present Modally
3. Popup
4. Show Detail (e.g.: Replace Segue)
1. Show (E.G.: Push Segue)
This Segue allows you to navigate back and forth through a pile of views that stack on top of each other. It’s indeed one of the most used segues.
Navigation Controller appears as a bar on top, usually with a title in the center and arrow in the left side. It controls the stack of views.
This pushes the next View Controller onto the navigation stack allowing the user to click the back button to return to the previous screen through the back button on the top left of the Navigation Bar.
2. Present Modally
This Segue shows a new view controller on top of the previous one.
A modal view controller is not supposed to be part of any stack of screens. It is just sits on top of the previous view controller.
This one does not need to be part of a UINavigationController or UISplitViewController. It is just sits on top of the previous view controller.
3. Popup
This Segue shows the new View Controller over the previous one in a smaller box, usually with an arrow pointing to which UI Element created the popover.
This type of segues is implement in an iPad.
When used on in iPhone, it is not much different than the “Present Modally” segue by default, but it can be configured to act more like an iPad.
Popup segues need an anchor. This anchor is the view where the arrow of the popup will point to the button. You want to your popup to point to the button or view that triggers it’s appearance.
4. Show Detail (E.G.: Replace Segue)
This segue is a special type of segue that’s useful for split view controllers.
In the split view controller, you have a “Master” view controller at the left that controls the “Detail” view controller at the right.
Else if it is not the master detail then the current content is replaced with the new one.
Segue is implement two methods
1. Declaration: Perform Segue(WithIdentifier: String, Sender: Any?)
Segue are initiated automatically and not using this method. However, you can use this method where the segue could not be configured in your storyboard file.
Parameters:
Identifier
The string that identifies the triggered segue. In Interface Builder, you specify the segue is identifier string in the attributes inspector.
This method is throws an Exception Handling if there is no segue with the specified identifier.
Sender
The object is that you want to use to initiate the segue.
This object is a made available for informational purposes during the actual segue.
2. Declaration: Prepare(For Segue: UI Story board Segue, Sender: Any?)
You can use prepare For Segue: method when pass any object one View Controller to another View Controller.
Parameters :
Segue
This object containing information about the view controllers involved in the segue.
Sender
The object that initiated the segue. You might use this parameter to perform different actions based on which control initiated the segue.
How To Implement Segue In Your Project ( Segue: 1 )
- Step - 1: Select the segue in your storyboard.
- Step - 2: Select the Attributes Inspector then set the segue identifier “navigationSegue”.
In this View Controller, you must have to add Text Field, Image View, button as per your need.
Now, Create UI Outlet of UI Text field, Imageview and Button.
@IBOutlet weak var txtEnterText: UITextField!
@IBOutlet weak var imageView: UIImageView!
@IBOutlet weak var btn_segue: UIButton!
Now, Create @UIAction for your UIButton. Same as bellow code.
// MARK:- Segue Navigation Button Action Event
// MARK:- Textfield Validation
// MARK: Instance Method
First view controller connected to Second view controller using performSegue(withIdentifier: String, sender: Any?) method through “navigationSegue” identifier in button action event.
Validation function is used to set required validation of textfield. But first set delegate method and protocol in your class.
One view controller to another view controller pass any type of object using reference object in prepare(for segue: UIStoryboardSegue, sender: Any?) instance method.
Home View Controller ( Segue: 1 )
Second View Controller ( Segue: 1 )
2. Push/Pop
Push Navigation is pass data between one view controller to another view controller without using any type of segue.
But it’s used to Storyboard id of destination view controller.
So, set Storyboard id of view controller.
How To Implement Push/ Pop In Your Project ( Push/Pop: 2 )
There are some simple steps and its necessary to add into your project:
- Step - 1: Select the initial view controller in your storyboard.
- Step - 2: Select the Identity Inspector of right side panel in your project.
- Step - 3: Assign Storyboard ID for reference view controller.
1. Declaration
pushViewController( _ viewController: UIViewController,animated:Bool)
Parameters:
viewController
The view controller to push onto the stack. This object is connectivity of destination view controller.
Animated
Set Bool true so view controller is animate the transition or set Bool false so view controller is not animate the transition.
Now, Create @UIAction for your UIButton. Same as bellow code in source view controller.
// MARK:- Push Navigation Button Action Event
Push view controller is implemented in source view controller. It’s pass any types of data between one view controller to another view controller.
In this code secondView object is connectivity of storyboard using storyboard identifier.
2. Declaration:
popViewController(animated : Bool )
Pop view controller navigation is used to jump to root view controller without any type of segue.
Parameters
Animated
Set Bool true so view controller is animate the transition or set Bool false so view controller is not animate the transition.
Now, Create @UIAction for your UIButton. Same as bellow code in destination view controller.
// MARK:- Pop Navigation Button Action Event
3. Present / Dismiss
Present is a view controller modally.
This method is gives up the current screen to another view controller.
The default presentation animation is simply slides to the new view up to cover the current one.
How To Implement Present/Dismiss In Your Project
There Are Some Simple Steps. And its Necessary to add into your project,
- Step - 1 : Select the initial view controller in your storyboard.
- Step - 2 : Select the Identity Inspector of right side panel in your project.
- Step - 3 : Assign Storyboard ID for reference view controller.
1. Declaration :
present( _ viewControllerToPresent : UIViewController, animated flag:
Bool,completion : (() -> Void)? = nil )
Parameters
viewControllerToPresent
The view controller to display over the current view controller is content.
Animated Flag
Set Bool true so view controller is animate the presentation or set Bool false so view controller is not animate the presentation.
Completion
The block to execute after the presentation finishes. This block has no return any value and takes no parameters. You may specify nil for this parameter.
Now, Create @UIAction for your UIButton. Same as bellow code in destination view controller.
// MARK:- Present Navigation Button Action Event
One view controller to another view controller pass any type of object using reference object in present (_:animated:completion:) instance method.
dismiss(animated flag: Bool, completion : (() -> Void)? = nil )
Parameters
Animated Flag
Set Bool true so view controller is animate the transition or set Bool false so view controller is not animate the transition.
Completion
This block to execute after the view controller is dismissed. This block has no return any value and takes no parameters. You may specify nil for this parameter.
// MARK:- Pop Navigation Button Action Event
@IBAction func btn_pop_navigation(_ sender: UIButton) { self.dismiss(animated: true, completion: nil) }
The presenting view controller is responsible for dismissing view controller it presented. If you call this method on the present view controller itself, UIKit asks the presenting view controller to handle the dismissal.
Final Code ( Home View Controller )
Import UI kit
// MARK:- Segue Navigation Button Action Event
// MARK:- Push Navigation Button Action Event
// MARK:- Present Navigation Button Action Event
// MARK:- Textfield Delegate
// MARK: Instance Method
Final Code ( Second View Controller )
Import UIKit
// MARK:- Pop Navigation Button Action Event
Final Code ( Constant View Controller )
Conclusion:
We usually need a navigation controller, to navigate from one view to another view controller of an iOS application.
In this blog, we have talked about navigation controller work from one screen to another in Swift. There are ready-to-use codes with examples for better understanding and ease in your work. And at last, presented a demo for you.